A Look at the BlackBerry OS as a Secure Platform for Third Party Applications
on 1 September, 2012
At MWR labs, we have recently been delving a little deeper into the security features of the BlackBerry OS and how secure this platform is for third party applications to operate in.
In this blog, we focus on the risks third party applications face and the ways in which developers can secure their applications from these risks. We will be looking at applications operating within the BlackBerry Internet Service (BIS) environment.
The BlackBerry OS has historically enjoyed a great reputation for being the most secure mobile platform, this is true for devices that are locked down operating in a BlackBerry Enterprise Server (BES) environment. However, we are focusing on third party applications running on a consumer’s device running in the BIS environment and have come across some interesting findings! These findings should be of particular interest to developers who create consumer applications for BlackBerry and to security consultants looking to test BlackBerry applications.
Storage of Data
First, let’s focus on the storage of data on the device. BlackBerry OS differs in how applications store their data compared with other mobile platforms such as Android. With BlackBerry, there is no default protection built in, and there is no protected storage area. Instead, applications have to protect their data manually using a code-signing key unique to the developer.
Here comes the first problem, a lot of application developers are not aware of this and often leave their data completely unprotected. This problem is prevalent with developers that learnt their trade on other platforms where protection is the default.
The next issue is that relying on developers to protect their own data can often result in vulnerabilities that result from logical mistakes and poor assumptions. We will illustrate just such an example with the following code, taken from the “MWR Legit App” proof of concept (available to download below), which is used to store data in a SQLite database.
URI myUri = URI.create(strDbPath);
// Check if database exists
fileCon = (FileConnection) Connector.open(strDbPath);
// If database exists add a record
if(fileCon.exists()){
// Open database
db = DatabaseFactory.open(myUri);
add(new LabelField("Database already exists"));
// Add data to the database
stat = db.createStatement("INSERT INTO Users(Id, User, Password) " +
"VALUES (1, 'John', 'secret')");
stat.prepare();
stat.execute();
add(new LabelField("Adding Data to DB"));
}
// If database doesn't exist create it
else{
// Get code signing key
CodeSigningKey codeSigningKey = CodeSigningKey.get(ApplicationDescriptor.currentApplicationDescriptor()
.getModuleHandle(), "ZAK");
// Create Database Security Options to ensure database is encrypted and protected
DatabaseSecurityOptions dbSecOpt = new DatabaseSecurityOptions(codeSigningKey);
// Create protected Database
db = DatabaseFactory.create(myUri, dbSecOpt);
// Create Table
stat = db.createStatement( "CREATE TABLE Users ( " +
"Id INTEGER, User TEXT, Password TEXT )" );
stat.prepare();
stat.execute();
add(new LabelField("Creating DB"));
// Add Record
stat = db.createStatement("INSERT INTO Users(Id, User, Password) " +
"VALUES (1, 'John', 'secret')");
stat.prepare();
stat.execute();
add(new LabelField("Adding Data to DB"));
}
fileCon.close();
stat.close();
db.close();
The code here is quite simple and it should be clear from the comments what is going on. The example is a little contrived in that it if the database does exist it just keeps adding the same data but this is for demonstration purposes.
If you haven’t spotted it, the vulnerability that exists here is that the application fails to check if the database that exists is protected with its code signing key. What would happen if a malicious application deleted the existing protected database and then created an empty unprotected database. Well, when the legitimate application loads up it will see that the database exists and then write data to it. The malicious application is then able to read this data as the database remains unprotected. The “MWR Malicious App” proof of concept does exactly that. Similar vulnerabilities can exist with the other storage types available on BlackBerry.
In order for a developer to protect themselves against these types of attacks, it is important that they check that the storage type they are using is protected each time before they read or write to the storage. The code below shows how this can be achieved with a database.
db = DatabaseFactory.open(myUri);
// Check to see if the database is protected with our code signing key
if(myCodeSigningKey != db.getDatabaseSecurityOptions().getCodeSigningKey()){
throw new Exception("Database is not protected!");
}
It is also worth noting that a malicious application could continually delete the database to perform a Denial of Service (DoS) attack. Developers should therefore ensure that their applications guards against this e.g. backing up data to a server.
Transmission of Data
An application that transmits sensitive data (e.g. usernames and passwords) wants to know that this data is secure and sent over a secure channel. This is normally achieved with HTTPS, however it is not that simple with BlackBerry due to the numerous different ways connections can be made. The following table shows the key different ways that an application can make a connection and MWR’s recommendations.
WiFi | Mobile Network | |
---|---|---|
End to End | Insecure | MWR Recommends |
Through BIS | Insecure | Insecure |
The meanings of WiFi and Mobile Network are self-explanatory, they are simply connections made over WiFi or over the mobile network. What is more interesting are connections made end-to-end or through BIS. An end-to-end connection is simply a connection that is made directly between the device and the remote server that the application wishes to communicate with. An HTTPS connection made through the BIS is different and is achieved through first connecting to the BIS. The diagram below shows how these connections are made.
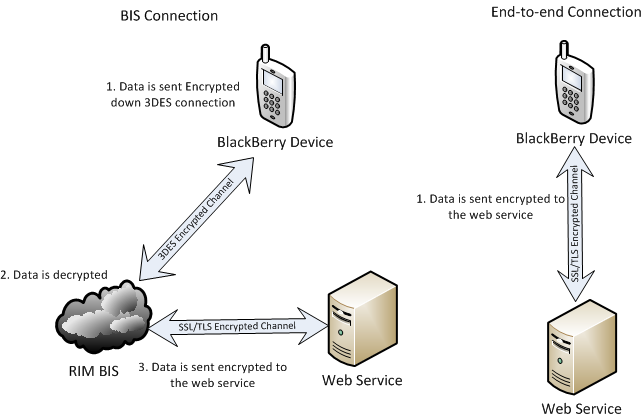
As we can see, the problem with making connections through the BIS is that the data sent will be unencrypted whilst it is in RIM’s network (between 2 and 3 in the diagram). By default, connections will be made like this, MWR recommend that connections are instead made end-to-end. Code below shows how this can be achieved.
We have also flagged up WiFi as being insecure. There is a simple reason for this, there is no way for an application to determine whether or not a valid SSL certificate is being used. If the web server has an invalid SSL certificate then the user will be prompted with the option of trusting the certificate. However, this happens completely transparently to the application. An application can only find very limited information about the certificate being used such as Issuer Name, Common Name and Serial Number all of which can easily be spoofed. Such behaviour contrasts with say Android applications, where a user would have to manually add a certificate into the Android certificate store in order to trust an invalid certificate. Additionally on Android it is possible for an application to check the signature of a certificate.
This behaviour puts BlackBerry applications at a greater risk to Man-in-the-Middle attacks (MitM). This is why MWR recommend that connections are made through the mobile network as it is significantly harder to perform MitM attacks over the mobile network compared to WiFi. Below is code that shows how a secure connection can be made that is both end-to-end and over the mobile network.
The following code demonstrates how to make a connection as securely as possible:
// Use connection factory
ConnectionFactory connFact = new ConnectionFactory();
// Set WiFi as a banned type
int bannedTypes[] = new int[1];
bannedTypes[0] = TransportInfo.TRANSPORT_TCP_WIFI;
connFact.setDisallowedTransportTypes(bannedTypes);
// Make the connection and make it end to end
ConnectionDescriptor connDesc;
connDesc = connFact.getConnection("https://labs.mwrinfosecurity.com;EndToEndRequired");
HttpsConnection httpsConn;
httpsConn = (HttpsConnection)connDesc.getConnection();
The Application Sandbox or Lack off
Now we are going to turn our attention to the ways an application can be interacted with by a malicious application. There are two key concerns here, screenshots and event injection or input simulation.
Event Injection
Event Injection, also known as input simulation, allows an application to simulate key presses, touch events and track pad movements. This functionality was perhaps included to allow applications to perform automated testing. The key problem with event injection is that an application can perform event injection on other applications. However, event injection is set to deny by default. At this point it is worth looking at what that means, and what the other BlackBerry permissions are. The following table highlights this.
Permission State | Description |
---|---|
Allow | The application can use this permission |
Prompt | The user is prompted every time the application attempts to use this permission |
Deny | The application cannot use this permission* |
* Unless the application specifically requests that permission, in which case just prompt the user!
As we can see there really is no difference between Prompt and Deny and so while this dangerous permission is set to deny by default, a malicious application can request this permission from the user. Since BlackBerry 6, permissions have been put into groups, the input simulation permission lies in the advanced capability group. It wouldn’t be too hard to convince the user that the application is very advanced and thus needs the advanced capabilities permission to run.
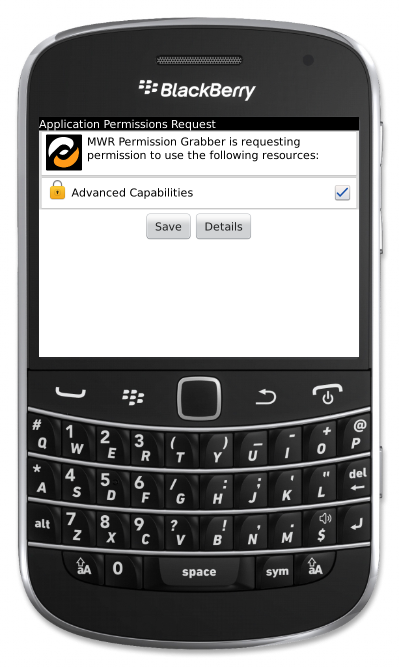
A malicious application could use this functionality to perform actions to a legitimate application. Such an attack could be carried out as follows:
- Have the malicious application run in the background on start-up
- Wait until the user has been idle for a while
- Launch the application you want to exploit
- Quickly perform input events (your payload)
- Lock the device or similar
The ways in which applications could be exploited by this are application specific. One example would be an auction application. The malicious application could launch this application and then bid on the attacker’s item.
Another interesting attack vector pointed out here1 is the fact that input injection can be used to get other permissions. A malicious application simply requests another permission from the BlackBerry OS, forcing a prompt to be displayed, the malicious application then quickly simulates the input required to accept this permission.
The proof of concept application “MWR Permission Grabber”, available to download at the bottom, does exactly this. Once this application has been granted the advanced capabilities permission it can grant itself any other permission without any user interaction. Please note that you do need to interact with the application to specify what permission it should grab!
Currently there is no known remedial action for preventing input simulation in your application in the BIS environment. Mitigation would involve requiring a user to enter their password before performing sensitive functionality.
Screenshots
Another instance of where an application can do malicious things whilst running in the background is with screen shots. There is a simple API called Display.Screenshot() that allows an application to take a screenshot no matter what is currently being displayed on the device. The recording permission is required in order to do this, however, see above for how this can be acquired! This is in contrast to other mobile platforms, with Android there is no way for an application to take a screenshot unless the application has root access.
This can be a problem wherever an application displays sensitive data as an application running in the background will always be able to capture that data in a screenshot. Think of that important company memo you are reading, you may not be the only one reading it!
You can see this in action, the proof of concept application “Screen Grabber” will take screenshots at a specified interval and store them in the gallery. Again, there is currently no remedial action for preventing screenshots of your application from being taken in the BIS environment.
Having discussed input simulation and screenshots, you may have thought of an interesting idea, got it? Yes, that’s right! A malicious application could allow a remote attacker to take full control of a BlackBerry! The application could send screenshots to a server so that the attacker can see the screen. The attacker could then send input to the application, which would use event injection to perform that input on the device. Think of VNC but for BlackBerry. It should be noted that the only permission required is the advanced capabilities permission as the screen capture permission can be acquired using event injection as mentioned above.
Summary
The BlackBerry OS may not be as secure as you thought, check the list!
- Data storage is unprotected by default, which often results in logic errors
- There are issues related to securely transmitting data
- Applications can escalate their permissions
- Malicious applications can take full control of your application!
Because of these issues, great care has to be taken when developing applications on BlackBerry. Developers need to be aware of the possible attacks their applications could come under and defend themselves against them.
Finally, it is worth reiterating that these are issues faced by a consumer application that will operate on a consumer’s device in the BIS environment. A BES environment correctly locked down would not face the same issues.
Proof of Concept Applications
The following proof of concept applications have been created to demonstrate the issues highlighted above and allow people to witness these issues first-hand. These applications have been tested and known to work on a BlackBerry Curve 9380. They have also been tested on different models using the BlackBerry Simulator. The applications are available to download as COD files, these are BlackBerry binary files. They can be installed using the javaloader program which is bundled with the BlackBerry SDK or available to download separately below.
Download: JavaLoader
MD5: 55E4FCD8AD4FF5874110443074F6338D
SHA-1: EE9DA551A4AEFB8C89CC66991EAC93A07F186C1A
Legit Signed Application
Download: MWR_Legit_App
MD5: D841E6BCD4E944FD53ACCDFFA431B9E3
SHA-1: 7AEB038F1647257681785F46EAB6C657BEFF2F31
The Legit Signed Application creates an encrypted and protected database if the database doesn’t exist, or adds data to the database if it already exists. This application can create the database either in the store or on the SD card.
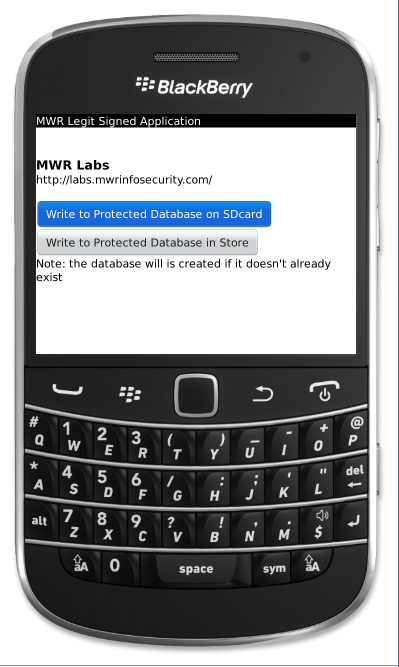
Malicious App
Download: MWR_Malicious_App
MD5: 78BB2221BE45AB835545B5136415A5C5
SHA-1: D8D86B4B82151DC60FC1816EFA04CFD1E11FEA2C
The Malicious Application will delete any protected database created by the legitimate application and then create an empty unprotected database. To do this just hit the relevant “Create Empty Unprotected DB” button. The database can then be dumped by hitting the relevant “Dump DB” button. If the legitimate application writes any data to the database the malicious application will now be able to read this data.
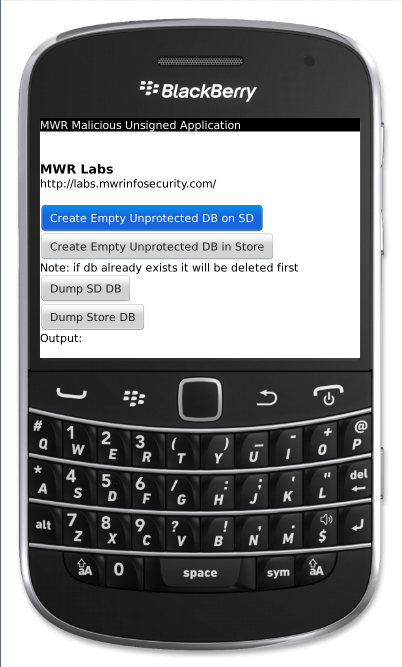
Permission Grabber
Download: PermisionGrabber
MD5: BF60C441DDD5CD6258A9D880AC9E7EB1
SHA-1: F9C4C04F326329062D3DE36BBE177E35B005D280
The Permission Grabber application uses input simulation to grant itself access to additional permissions. A list of the application’s current permissions can be obtained by hitting “Value Of All Permissions”. To grant the application a specific permission, select the permission, select the correct OS version and then hit “Get Selected Permission”. The delay time is the time in milliseconds after the permission is requested before input simulation is performed. The sleep time is time in milliseconds to wait between simulated inputs. These can be altered to see how fast permissions can be granted.
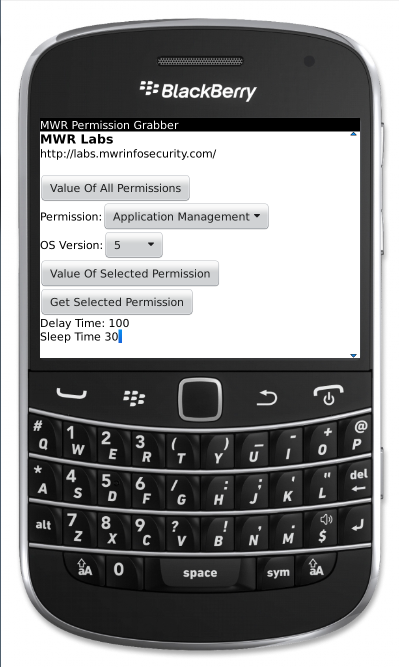
Screen Grabber
Download: MWRScreenGrabber
MD5: EAF393A8638980D56B28D09550371EB1
SHA-1: BB2CB8DBFE20372BE01B378B9E682E67CB4C29D4
The Screen Grabber application will take screenshots in the background and save them to the gallery. Simply specify the interval for which to take screenshots and hit start. To stop taking screenshots navigate back to the application and hit stop.
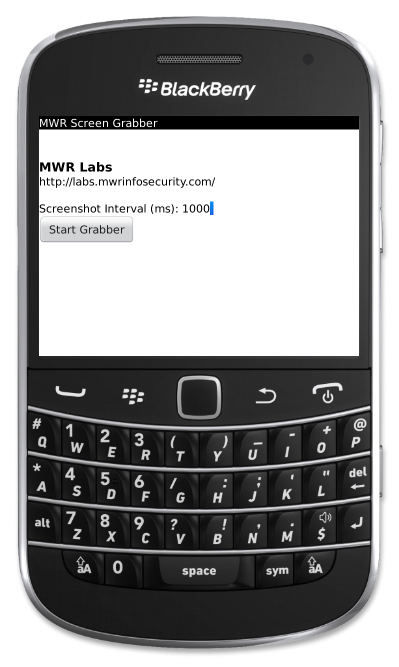
References
1 http://www.kulkan.com/en/proyectos_blackberry_security/abusando-de-la-simulacion-de-entrada/